Embarking on the journey of server-side development, Node.js emerges as a formidable JavaScript runtime environment. For those aiming to install Node.js on Debian 12 Bookworm, Debian 11 Bullseye, or Debian 10 Buster, understanding Node.js’s essence is crucial. This guide will first cover the core features of Node.js.
Node.js Highlights:
- Asynchronous Architecture: Node.js thrives on an event-driven, asynchronous framework, adeptly managing multiple requests concurrently, enhancing performance and scalability.
- Efficient I/O Model: Its non-blocking I/O model ensures swift data processing, even when juggling multiple connections, optimizing responsiveness.
- Modular Design: Embracing modularity, Node.js facilitates easy code creation, management, and reuse. With the support of the CommonJS module system, developers can tap into the vast reservoir of third-party modules via the Node Package Manager (npm).
- Broad Compatibility: Node.js’s adaptability spans macOS, Linux, and Windows, catering to various development environments.
- Robust Ecosystem: A vibrant community continually enriches Node.js’s ecosystem, offering a plethora of libraries, frameworks, and tools that streamline the development journey.
With a grasp of Node.js’s distinctive attributes, integration is the next step. This guide will detail how to install Node.js on Debian 12 Bookworm, Debian 11 Bullseye, or Debian 10 Buster, leveraging the NodeSource third-party repository. This method ensures access to the latest Node.js versions, the most recent stable or LTS release.
Install Node.js on Debian 12, 11, or 10 via DEFAULT APT
This section will review how to install Node.js directly from the Debian repository. This method is straightforward but might not provide the latest version of Node.js.
Step 1: Update Package List Before Node.js Installation
Before installing Node.js, updating the package list is essential to ensure you get the most recent version in the repository. Run the following command:
sudo apt update
This command will update the package list and provide information about any available updates for your system.
Step 2: Install Node.js via APT Command
Now that your package list is updated, you can install Node.js using the following command:
sudo apt install nodejs
This command will install Node.js along with its required dependencies. Once the installation is complete, you can check the installed version by running:
nodejs -v
This will display the installed version of Node.js on your system.
Install Node.js on Debian 12, 11, or 10 via NodeSource
If you want to install a more recent or custom version of Node.js, you can use the NodeSource PPA. This method allows you to choose a specific version of Node.js and ensures you’re getting the latest release.
Step 1: Install Required Initial Packages
Begin by opening your terminal and executing the following command. This installs essential packages needed for Node.js installation from NodeSource on Debian:
sudo apt install curl apt-transport-http ca-certificates
Step 2: Import NodeSource
Initiate the Node.js setup by importing the NodeSource repository’s GPG key. This step ensures the legitimacy of the packages to be installed. Enter this command:
curl -fsSL https://deb.nodesource.com/gpgkey/nodesource-repo.gpg.key | gpg --dearmor -o /usr/share/keyrings/nodesource.gpg
Next, determine the specific Node.js version for your Debian system. For instance, change NODE_MAJOR=20
to your preferred version, like NODE_MAJOR=18
. Execute:
echo "deb [signed-by=/usr/share/keyrings/nodesource.gpg] https://deb.nodesource.com/node_$NODE_MAJOR.x nodistro main" | tee /etc/apt/sources.list.d/nodesource.list
Node.js version options include:
NODE_MAJOR=16
NODE_MAJOR=18
NODE_MAJOR=20
NODE_MAJOR=21
Step 3: Install Node.js via NodeSource APT
With the NodeSource repository in place, proceed to install Node.js using the command below. This approach provides the most up-to-date or specific Node.js version, enhancing Debian beyond the standard repository offerings:
sudo apt install nodejs
This command ensures the installation of Node.js along with all required dependencies, tailored to your selected version.
Finally, confirm the installation of Node.js:
node --version
The displayed version number will verify the successful installation of Node.js on your Debian system.
Install Node.js on Debian 12, 11, or 10 via NVM
Another way to install Node.js is using the Node Version Manager (NVM). This method allows you to manage multiple Node.js versions on your system, making switching between versions for different projects easier.
Step 1: Install NVM on Debian
To install NVM, run the following command:
curl -o- https://raw.githubusercontent.com/nvm-sh/nvm/master/install.sh | bash
or
wget -qO- https://raw.githubusercontent.com/nvm-sh/nvm/master/install.sh | bash
This command downloads and runs the NVM installation script. Once the installation is complete, you need to restart your terminal or run the following command to load NVM:
source ~/.bashrc
Step 2: Install Node.js via NVM Command on Debian
In this step, we’ll install Node.js using the Node Version Manager (NVM). NVM allows you to manage multiple Node.js versions on your system, making switching between versions for different projects convenient.
List available Node.js versions
Before installing a specific version of Node.js, you can check the available versions by running:
nvm ls-remote
This command will display a list of all available Node.js versions. It helps you identify the version you want to install, such as the latest LTS release or a specific version number.
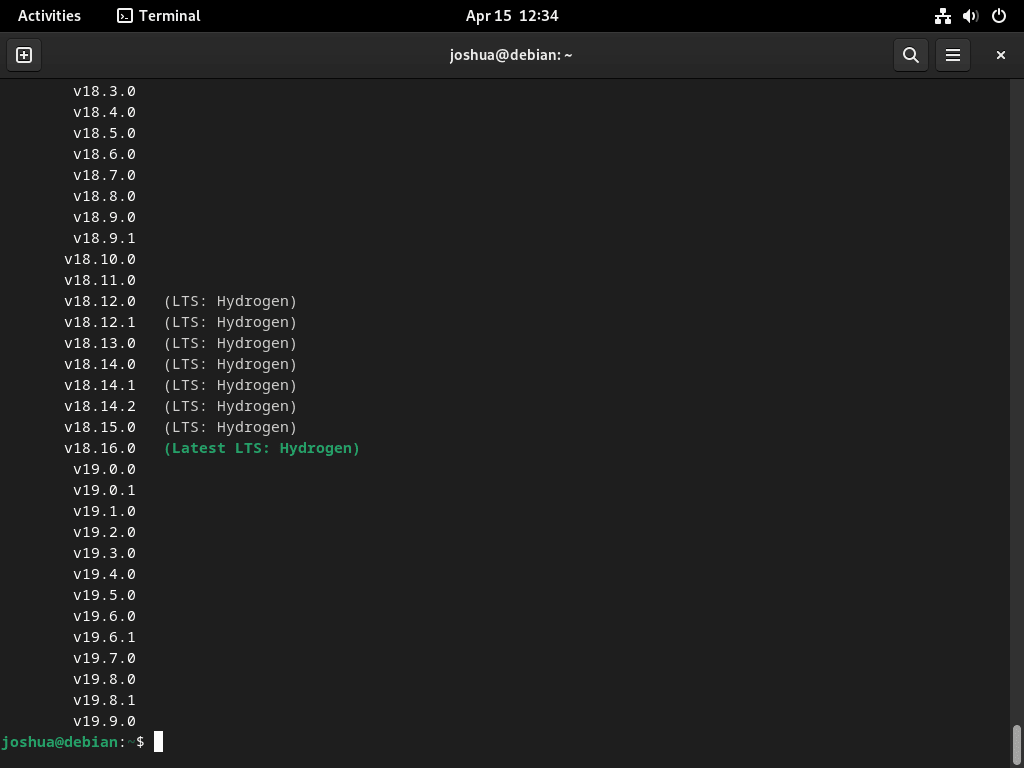
Install a Node.js version via NVM Command
With NVM installed, you can now install the desired version of Node.js by running the following:
nvm install <version>
Replace <version>
with the specific version you want to install. For example, if you want to install Node.js version 19.9.0, you would run:
nvm install 19.9.0
This command will download and install the specified version of Node.js.
Verify Node.js installation
To check the installed version of Node.js, run the following:
node -v
This will display the installed version of Node.js on your system.
Switch between installed Node.js versions
NVM allows you to switch between different Node.js versions easily. To switch between installed Node.js versions, use the following command:
nvm use <version>
Replace <version>
with the version you want to switch to. For example, if you want to switch to Node.js version 18.16.0, you would run:
nvm use 18.16.0
This command will set the specified version as the active Node.js version for your current session. To make a specific Node.js version the default for new terminal sessions, use the command:
nvm alias default <version>
Replace <version>
with the desired version number. For example, to set Node.js version 18.16.0 as the default, you would run the following:
nvm alias default 18.16.0
You have successfully installed and learned how to manage Node.js versions using NVM.
Additional Node.js Commands on Debian 12, 11 or 10
Remove Node.js From Debian
Remove Node.js installed from the Debian Repository or PPA
If you installed Node.js from the Debian repository or a PPA, you can uninstall it using the apt
program. First, let’s explain the command:
sudo apt remove nodejs
This command will remove Node.js along with its associated configuration files. It will prompt you to confirm the removal, and after confirmation, it will proceed with the uninstallation.
Remove Uninstall Node.js installed via NVM
If you installed Node.js using the Node Version Manager (NVM), you can uninstall it by following these steps:
First, check the current version of Node.js installed by running the command:
nvm current
This command will display the active Node.js version on your system.
Before uninstalling the current version of Node.js, you need to deactivate NVM by running:
nvm deactivate
This command will unload the active Node.js version from your current session.
Now, run the following command to uninstall a specific version of Node.js installed using NVM:
nvm uninstall <version>
Replace <version>
with the version number you want to uninstall. For example, if you want to uninstall Node.js version 19.9.0, you would run:
nvm uninstall 19.9.0
This command will remove the specified Node.js version from your system.
Conclusion
In summary, there are multiple ways to install Node.js on a Debian Linux system. You can install Node.js from the Debian repository using the NodeSource PPA or the Node Version Manager (NVM), depending on your requirements. Each method has its advantages, with NVM offering the most flexibility for managing multiple Node.js versions on your system. As your development journey progresses, you can choose the installation method that best suits your needs, ensuring a seamless and efficient workflow.
Big typo in “Step2: Import NodeSource APT Repository”!
Correct step is:
curl -fsSL https://deb.nodesource.com/gpgkey/nodesource-repo.gpg.key | gpg –dearmor -o /usr/share/keyrings/nodesource.gpg
NODE_MAJOR=20
echo “deb [signed-by=/usr/share/keyrings/nodesource.gpg] https://deb.nodesource.com/node_$NODE_MAJOR.x nodistro main” | tee /etc/apt/sources.list.d/nodesource.list
thanks for pointing this out, i am going to revert back to the installation scripts again as i removed them not that long ago.
unless the maintainers change their mind again.
Yes I have tried NVM. It’s says using node v20 after installation through NVM but then when I type the command node –version It says no such directory exist.
I too don’t know the exact reason may be it’s due to raspberry pi being used on VM not sure though. I and this person faced the same issue https://forums.raspberrypi.com/viewtopic.php?t=358959
Hey, I am using debian 11 on virtual machine and OS is raspberry pi desktop OS. But I can’t install latest node on it. I have tried all methods but it always installs node 12 which is obsolete now. Can you help with it? I followed the https://github.com/nodesource/distributions commands but that too didn’t worked.
Any help in this regard will be appricaited, Thankyou.
Hi Javeria,
Did you try NVM? i just installed this fine on both AMD64 and ARM64 for Node 12, infact installed a few of the minor versions of it.
While it wasn’t Raspberry PI, it was Debian 11 for both architectures.
Let me know, I would be curious why this would not work. NVM is the king for working with older versions as a developer.
Maybe I have not explained something properly enough, let me know either way.
Thanks.